Lecture 1, ACM 11
Contents
- When to use MATLAB
- Getting started
- Basics: using MATLAB as a calculator (this is an example of a cell)
- using a simple variable
- vectors and matrices
- precautions about matrices
- Strings, and such; use single quotes always!
- Breaking up long-lines of code onto two lines
- very simple plotting
- using the HELP menu
- and finally, the powerful Help Browser: either hit "F1" key, or...
- "help" also works on directories
- More sources of help
- More on matrices and vectors
- "for" loops
- linear systems
- about this file
When to use MATLAB
%{ These notes will be posted on the class website First topic: which language to use, and when MATLAB excels at Numerical Computation. It is also easy to use, and is a very high-level language (for example, you can multiply two matrices with a single command). MATLAB competes with many languages, including: - Mathematica, Maple and other Sybolic math packages - MATLAB imitations (FreeMat, Octave, SciLab, xmath) and IDL, mathCad, etc. - C and Fortran [staples of the high-performance community] - C++, as well as C# etc. [modern Object-Oriented languages] - java and python [also modern Object-Oriented languages] - perl, bash and other shells [mainly data manipulation] - Microsoft Excel [data manipulation, plotting] - S and R [statistics languages] When to use MATLAB? (1) if you can afford to buy it, or can get it free (2)(a) for numerically intensive computations, or... (2)(b) for plotting and dealing with data (3) for faster DEVELOPMENT than C and Fortran (4) for faster EXECUTION than most other languages When NOT to use MATLAB? (1) for symbolic math, if you have access to Mathematica or Maple (2) you want free software, or want to release code that doesn't require the user to have MATLAB (3) you are doing something SERIOUS, like climate modelling, solving 3D Partial Differential Equations, etc. Most languages can do everything, even if they are not the best at it. Here are some compelling reasons to use MATLAB: (1) the language is rather fast to learn, and many functions are already builtin (2) the HELP menu is fantastic (3) MATLAB deals with sparse matrices very well (4) the debugger and profiler are powerful and easy to use (5) MATLAB is an industry standard, much like Microsoft Word, or autocad. This also means that the web is full of MATLAB resources. MATLAB is not a compiled language. It is also much more than a language, since it provides many graphical tools as well as extended functionality with toolboxes. %}
Getting started
%{ Basics: development environment - command prompt (note: MATLAB can be run from the terminal, more on this later) You can use tab-completion, and use the up-arrows. Drag-n-drop also works. - m-file editor (note: you can use your own editor too) - Command History window - Workspace - for plotting, and Variable Editor - Current Directory, and file browser - Menus, and links to the Help Menu - when you plot a figure, the Figure menus are also available (more on plotting later) All of these windows can be re-arranged What is this file? This is an example of a "m-file". The file is saved as "lecture1.m" MATLAB also automatically makes backup files, called either "lecture1.asv" (windows) or "lecture1.m~" (unix/linux); this behavior can be changed. m-files contain sequences of commands that are executed in order. It is very similar to using the command line, but much more convenient Two important concepts: (1) Comments. Everything written here is a comment. There are two ways to write comments: either comment a single line with "%", or use block comments to comment several lines, using "%{" followedd by "%}". See this file for examples. Note that you cannot have ANYTHING on the line that starts with "%{". By default, the editor puts comments in green (you can change this). For printing an m-file, you can make the comments appear in italics; please do this for your homework! See the "page setup" menu. (2) Cells. MATLAB has two meanings for the word "cell", so don't get confused. The first meaning of "cell" is used in a m-file, and it is a way to split the m-file into chunks, each of which can be executed separately. This is a very useful tool. To execute a single cell, see the button in the toolbar, or hold down "ctrl" and then hit "enter" on your keyboard. The beginning of a cell is a special comment line that begins with two "%", not just one. The other use of the word "cell" refers to a type of variable, and will be discussed later. Other basics The editor is highly customizable, and it has many keyboard shortcuts (most shortcuts are either the same as Emacs, or as basic Windows shortcuts). These can be customized as well. For your system, you should know the following shortcuts: (1) saving the file (e.g. ctrl+X, ctrl+S for unix, or cntr+S for windows) (2) commenting a line of code (e.g. ctrl+/ for unix, ctrl+R for windows) (3) uncommenting a line of code (e.g. ctrl+T for unix and windows) see the "Text" menu for more (e.g. changing the indent: ctrl+I) %}
Before we start, a brief check of the version number The requirements for this class are version 7.2 (R2006a) or later Please see instructor or TA with installation questions. Remember, for Windows Vista, run everything as Administrator. MATLAB is also on all Windows library computers, as well as on the ugcs cluster and the its machines. You can run MATLAB remotely as well.
clc version license('test','statistics_toolbox') clear % in MATLAB, like many languages, "1" means true and "0" means false % and you can also use the keyword "true" (without quotes) to make % your code more readable. For a general number n, n is considered "false" % if it is zero; any other value is "true".
ans = 7.2.0.294 (R2006a) ans = 1
Basics: using MATLAB as a calculator (this is an example of a cell)
(aka: why you will never use your TI-89 again)
clc % this clears the screen of old output
3 + 5
ans = 8
clc 3 + 5; % the semicolon suppresses output ( THIS IS A COMMENT) 3+ 5; % MATLAB ignores whitespaces usually % (though sometimes it treats them as a comma) % Note: comments can start ANYWHERE in a line of code % but you cannot "undo" a comment on the same line, e.g. % comment % code
using a simple variable
x = 4 % Note: we do not need to "declare" x, as we would in C or Fortran % MATLAB is case-sensitive, so if we as for the value of "X", we'll % get an error. % For naming your own variables, you can use any mixture of upper- and % lower-case. Variables may not start with a number, but can have % numbers elsewhere in the name.
x = 4
x = 8; 2*x % the semicolon suppresses output AND allows us % to add a second command.
ans = 16
clc
exp(i*pi) % "i" and "j" are both sqrt(-1)
ans = -1.0000 + 0.0000i
i = 5; exp(i*pi)
ans = 6.6356e+06
clear i % erases "i" from the workspace; it will now be sqrt(-1) again % Note: to clear more than one variable, do NOT separate the variable % names with a comma -- just uses spaces, e.g. % >> clear i j
clc x = exp( sin( atan( abs( log( 3 ) ) ) ) ); disp(x) % order of operations: don't memorize this, just use parenthesis % simplest commands: * (multiplication), + (addition), - (subtraction), % / (divistion), ^ (exponentiation)
2.0949
vectors and matrices
clc; x = [ 1 2 3; 4, 5, 6] % the spaces and commas separate columns % and the semicolons separate rows
x = 1 2 3 4 5 6
various ways to make a matrix
x = [ 1 2 3
5 4 6]
% in this case, the second line indicates a new row
x = 1 2 3 5 4 6
precautions about matrices
some operations are "matrix" operations, and others are "component-wise" aka "element-wise"
% x * x % this tries to do matrix multiplication
x .* x % this works element-wise
ans = 1 4 9 25 16 36
x = [1 2; 3 4]; exp(x) % this is element-wise expm(x) % this is an inherent matrix operation
ans = 2.7183 7.3891 20.0855 54.5982 ans = 51.9690 74.7366 112.1048 164.0738
x ^ 2 % this is matrix multiplication, e.g. "x * x" x .^ 2 % this is element-wise, e.g. "x .* x"
ans = 7 10 15 22 ans = 1 4 9 16
Strings, and such; use single quotes always!
Strings are matrices of characters; each character is represented by an integer (most common characters are represented by ASCII code, with an integer between 0 and 127)
clc; s = ' I am a ''string'
s = I am a 'string
s + 0 % automatic conversion to ASCII code ("double(s)" does the same)
ans = Columns 1 through 11 32 73 32 97 109 32 97 32 39 115 116 Columns 12 through 15 114 105 110 103
double('a string')
ans = 97 32 115 116 114 105 110 103
double( a string ) % this fails
char( s + 0 ) % convert back to characters char( s + 'A' - 'a' )
ans = I am a 'string ans = ) AM A STRING
Breaking up long-lines of code onto two lines
s2 = ['This is a long, boring, and seemingly pointless string that is' ... ' in fact so long, it must be written on two lines']; disp(s2)
This is a long, boring, and seemingly pointless string that is in fact so long, it must be written on two lines
very simple plotting
clc; ezplot( 'sin' ) % default domain is -2*pi to 2*pi
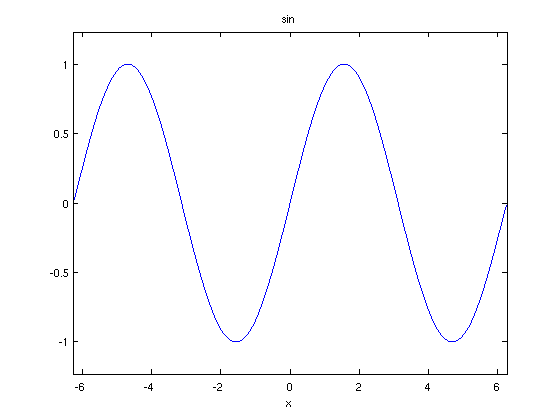
ezplot( 'cos(5*x)' ) % this time, MATLAB guesses that "x" is the variable
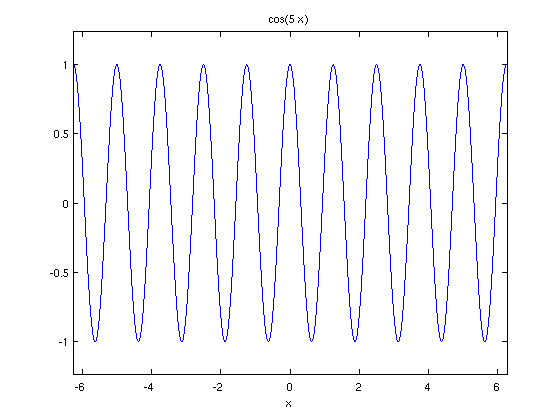
using the HELP menu
help ezplot %{ conventions: MATLAB functions are always lowercase. But, just to mess with you, the Help files always write them in all uppercase. Don't fall for it! %}
EZPLOT Easy to use function plotter EZPLOT(FUN) plots the function FUN(X) over the default domain -2*PI < X < 2*PI. EZPLOT(FUN2) plots the implicitly defined function FUN2(X,Y) = 0 over the default domain -2*PI < X < 2*PI and -2*PI < Y < 2*PI. EZPLOT(FUN,[A,B]) plots FUN(X) over A < X < B. EZPLOT(FUN2,[A,B]) plots FUN2(X,Y) = 0 over A < X < B and A < Y < B. EZPLOT(FUN2,[XMIN,XMAX,YMIN,YMAX]) plots FUN2(X,Y) = 0 over XMIN < X < XMAX and YMIN < Y < YMAX. EZPLOT(FUNX,FUNY) plots the parametrically defined planar curve FUNX(T) and FUNY(T) over the default domain 0 < T < 2*PI. EZPLOT(FUNX,FUNY,[TMIN,TMAX]) plots FUNX(T) and FUNY(T) over TMIN < T < TMAX. EZPLOT(FUN,[A,B],FIG), EZPLOT(FUN2,[XMIN,XMAX,YMIN,YMAX],FIG), or EZPLOT(FUNX,FUNY,[TMIN,TMAX],FIG) plots the function over the specified domain in the figure window FIG. EZPLOT(AX,...) plots into AX instead of GCA or FIG. H = EZPLOT(...) returns handles to the plotted objects in H. Examples: The easiest way to express a function is via a string: ezplot('x^2 - 2*x + 1') One programming technique is to vectorize the string expression using the array operators .* (TIMES), ./ (RDIVIDE), .\ (LDIVIDE), .^ (POWER). This makes the algorithm more efficient since it can perform multiple function evaluations at once. ezplot('x.*y + x.^2 - y.^2 - 1') You may also use a function handle to an existing function. Function handles are more powerful and efficient than string expressions. ezplot(@humps) ezplot(@cos,@sin) EZPLOT plots the variables in string expressions alphabetically. subplot(1,2,1), ezplot('1./z - log(z) + log(-1+z) + t - 1') To avoid this ambiguity, specify the order with an anonymous function: subplot(1,2,2), ezplot(@(z,t)1./z - log(z) + log(-1+z) + t - 1) If your function has additional parameters, for example k in myfun: %-----------------------% function z = myfun(x,y,k) z = x.^k - y.^k - 1; %-----------------------% then you may use an anonymous function to specify that parameter: ezplot(@(x,y)myfun(x,y,2)) See also EZCONTOUR, EZCONTOURF, EZMESH, EZMESHC, EZPLOT3, EZPOLAR, EZSURF, EZSURFC, PLOT, VECTORIZE, FUNCTION_HANDLE. Overloaded functions or methods (ones with the same name in other directories) help sym/ezplot.m Reference page in Help browser doc ezplot
if you don't know the name, here's a primitive way to search >> lookfor plot
and finally, the powerful Help Browser: either hit "F1" key, or...
doc ezplot %{ convention: when using a command like "doc", there are three ways to call it (1) doc ezplot (2) doc 'ezplot' (3) doc('ezplot') but the followig will NOT work (4) doc(ezplot) because MATLAB thinks the argument is a variable for more details, see >> help syntax %}
Overloaded functions or methods (ones with the same name in other directories) doc fixedpoint/ezplot doc symbolic/ezplot
"help" also works on directories
%{ % here is a list of very useful categories/directories help ops % lists the basic operations, very useful for beginners help elfun % lists things like cosine, exp, log, etc. help elmat % basic matrix stuff help datafun % min, max, convolution, fft, etc. help demos % a list of demos help general % workspace, path, saving/loading files, ... help graph2d % plotting help helptools % a list of the HELP functions help lang % control flow (if, for, ...), errors, help strfun % dealing with strings % ===== some other useful, but slightly more advanced categories help datatypes % stuff about characters, strings, integers, etc. help codetools % editing, debugging, profiling, openvar, pathtool, publish help funfun % functions acting on functions, % e.g. minimization, integration, ODEs help graphing % more powerful 2- and 3-D plotting tools % see also >> help plottools % and >> help scribe help graph3d % 3D graphics help matfun % useful, powerful matrix utilities, e.g. % finding eigenvalues, solving linear equations help polyfun % polynomial tools, e.g. finding roots, interpolation help sparfun % tools for sparse matrices help specgraph % special graphics: pie charts, countour plots, % movies, images help timefun % time functions: date, clock, timing an operation (tic, toc) % ===== and less useful or more obscure categories help iofun % for using zip, loading Excel files, downloading webpages, % sending email, basic reading/writing of files, % controlling a serial port, ... help specfun % special functions (e.g. Bessel functions), dot product, % number theory (primes, factor, factorial, nchoosek) help audiovideo % controlling the speakers, playing video... help guide % for designing GUI's; see also >> help uitools help mcc % the MATLAB compiler, more on this later if we have time help winfun % Windows specific stuff, e.g. winopen %}
More sources of help
%{ The internet has about 10,000 MATLAB tutorials. Unfortunately, I don't have any particular ones to recommend. If I missed some basic syntax stuff, the Wikipedia page on MATLAB probably has it. Books: Cleve Moler (founder of MATLAB) has a book online for free. Also, I've reviewed about a dozen current MATLAB books. See the website for links and titles. %}
More on matrices and vectors
An easy way to generate a simple vector:
clc; x = 1:8
% x = linspace(1,8, 8) % does the same thing
x = 1 2 3 4 5 6 7 8
Transpose:
x'
ans = 1 2 3 4 5 6 7 8
or
x.' % What's the difference? x' is actually the complex conjugate transpose, % aka the adjoint. If you just want to "flip" a matrix, use "x.'" % If x has all real numbers, they are of course the same.
ans = 1 2 3 4 5 6 7 8
Indices: use ( ) to access an element, not [ ] For creating a matrix, use [ ] not ( ).
clc; x = [ 1 2; 3 4; 5 6]
x(2,1) % convention is x(row, column)
x = 1 2 3 4 5 6 ans = 3
We can also use "linear indices", with "column-major" order (like FORTRAN, unlike C and most other languages). Major note: MATLAB is 1-indexed, unlike C.
x(2) x(5) % to convert from subscripts (e.g. x(2,1) ) to linear indices (e.g. x(2) ), % MATLAB has the functions "ind2sub" and "sub2ind"
ans = 3 ans = 4
More powerfully, we can index many entries at once, using either subscripts or linear indices. Here, we use the ":" operator. There are several equivalent syntaxes
x(1:3, 1) % shows just the first column ( x(1:3,2) is 2nd column ) x( :, 1) % exact same x(1:end, 1) % exact same; the special keyword "end" refers to the last % entry % This kind of referencing is sometimes called "slicing" % Note that the conventions are different that Python's slices
ans = 1 3 5 ans = 1 3 5 ans = 1 3 5
clc; x
x( 3:5 ) % note that this "flattens" the matrix into a vector
x = 1 2 3 4 5 6 ans = 5 2 4
clc; x
x( 1:2:5 ) % the "2" means that we go from 1 to 5 in increments of 2
x = 1 2 3 4 5 6 ans = 1 5 4
1:3:12
ans = 1 4 7 10
clc; r = randn( 1, 8) % make a random matrix of size 1 x 8
n = length(r);
r(2:end)
r = Columns 1 through 6 -1.9216 1.0150 0.4626 1.2842 -0.2236 0.4502 Columns 7 through 8 -1.1370 -0.8466 ans = Columns 1 through 6 1.0150 0.4626 1.2842 -0.2236 0.4502 -1.1370 Column 7 -0.8466
clc; R = randn( 3,7) R( 2, 3:5 )
R = Columns 1 through 6 -0.3679 -0.2076 0.1287 -0.5168 -2.9215 -0.3202 0.8675 0.7143 -0.1943 -1.0023 0.0595 -0.9822 -0.6179 1.0655 -0.0769 0.8008 0.7880 -0.3062 Column 7 0.3333 1.2973 -0.1534 ans = -0.1943 -1.0023 0.0595
"for" loops
"for" loops are easy in MATLAB, but the syntax is different than most other programming languages.
clc v = (1:4).^3 ; % a row vector for i = v disp(['v(i) is: ', num2str( i ) ]); end
v(i) is: 1 v(i) is: 8 v(i) is: 27 v(i) is: 64
make sure it is a row vector otherwise we get unexpected results
clc for i = v' disp(['v(i) is: ', num2str( i(1) ) ]); end
v(i) is: 1
a more compact way to write a for loop (ex. time how long it takes to do 500 FFT's of length 1000)
tic; for i = 1:500, fft( ones(1000,1) ); end; toc
Elapsed time is 0.028924 seconds.
linear systems
the backslash operator is also known as "mldivide" (this is useful if you want to see its "help" entry; see also "help slash")
clc A = hadamard(8) x = ones(8,1) b = A*x; xhat = A\b % solves the equation A*x = b for x % another way to solve: xhat = linsolve(A,b)
A = 1 1 1 1 1 1 1 1 1 -1 1 -1 1 -1 1 -1 1 1 -1 -1 1 1 -1 -1 1 -1 -1 1 1 -1 -1 1 1 1 1 1 -1 -1 -1 -1 1 -1 1 -1 -1 1 -1 1 1 1 -1 -1 -1 -1 1 1 1 -1 -1 1 -1 1 1 -1 x = 1 1 1 1 1 1 1 1 xhat = 1 1 1 1 1 1 1 1 xhat = 1 1 1 1 1 1 1 1
about this file
%{ This file was generated directly from lecture1.m using MATLAB's report generator. To use this, go to the File menu and select Publish (or "Save and Publish"); you can choose from several formats, including html. %}